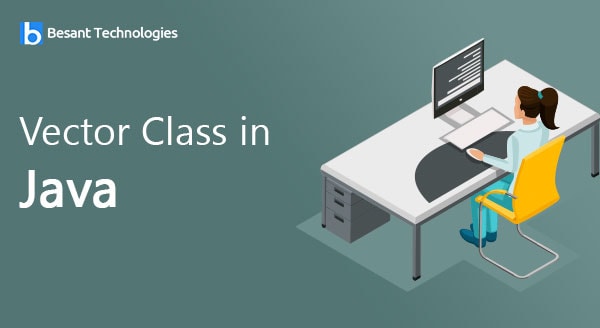
Vector Class in Java
Vector Class in Java
- Vector class implements List
- It uses Array data structure to represent the elements.
- Vector class is synchronized ( thread safe) unlike ArrayList class.
Various Syntax of Vectors :
Type | Syntax |
Non Generic Signature | Vector obj = new Vector(); |
Generic Signature | Vector <DataType> obj = new Vector<DataType>(); |
With Initial Capacity | Vector obj = new Vector(int initialCapacity); |
With Initial Capacity and Increment : | Vector obj = new Vector(int initialcapacity, int capacityIncrement ); |
Note : By default, the capacity of the vector is 10.
Example:
package mypackage; import java.util.Vector; public class VectorExample{ public static void main(String[] args) { Vector<String> vec= new Vector<String>(); vec.add("Happy"); vec.add("For"); vec.add(2, "ever"); //adding “i” in the index 1 System.out.println("*********************************************"); System.out.println("Vector elements are:"); for(String s:vec){ //iterating using for loop System.out.println(s); } System.out.println("The capacity of Vector-->" + vec.capacity()); System.out.println("The Size of Vector before Removal-->" + vec.size()); System.out.println("vector before Remval:"+ vec); vec.remove("For"); System.out.println("Vector after removal :" + vec); System.out.println("The Size of Vector after Removal-->" + vec.size()); System.out.println("*********************************************"); } }
Output:
*********************************************
Vector elements are:
Happy
For
ever
The capacity of Vector–>10
The Size of Vector before Removal–>3
vector before Remval:[Happy, For, ever]
Vector after removal :[Happy, ever]
The Size of Vector after Removal–>2
*********************************************
Click Here-> Get Prepared for Java Interviews
Methods of Vector Class :
Method Name | Description |
boolean add(E e) | Appends the element e to the end of the list. |
void add(int i, E element) | Inserts the element e at the specified index ‘i’ in the list. |
void addElement(E obj) | Inserts the element to the vector and increases the vector size by 1. This method is synchronised. |
int capacity() | Returns the current capacity of the vector.- default is 10 |
boolean contains(Object o) | Returns true if the list contains the element. |
E elementAt(int index) | Returns the element at given index. |
boolean isEmpty() | Returns true if the list has no elements |
void ensureCapacity(int minCapacity) | Ensures that the specified capacity is maintained. Increases the capacity if needed. |
void insertElementAt(Object obj, int index) | Inserts the object ‘obj’ in the vector at the specified index |
E remove(int index) | Removes the element at the specified index in the vector. |
boolean remove(Object o) | Removes the first occurrence of the object from the vector. |
void removeElementAt(int index) | Removes the element specified in the given index. |
int size() | Returns the number of elements in the list. |
E set(int index, E element) | Replaces the element at the specified index in the vector with the given element. |
boolean addAll(Collection<? extends E> c) | Appends all of the elements in the specified collection to the end of the vector. |
boolean removeAll(Collection<?> c) | Removes all the elements that are contained in the specified collection from the vector. |
Click Here-> Get Java Training with Real-time Projects