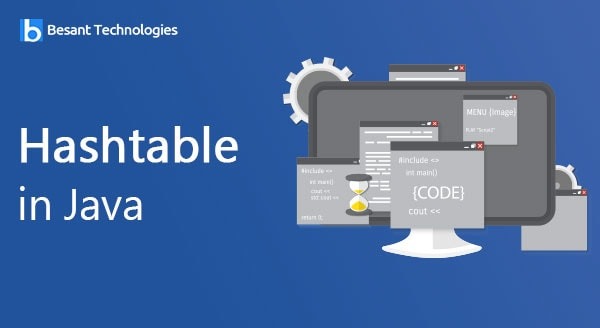
Hashtable in Java
Hashtable in Java
- Java Hashtable class implements a Map Interface and extends Dictionary class
- It is dual value pair collection in Java which stores <key,Value> pair in collection
The important points about Java Hashtable class are:
- A Hashtable is an array of list. Each list is known as a bucket. The position of the bucket is identified by calling the hashcode() method.
- A Hashtable contains values based on the key.
- It contains only unique elements.
- Value and key should not be NULL.
- It is synchronized. (Thread safe, can be used in multi-threading environment).
Syntax
Hashtable<Integer,String> hm=new Hashtable<Integer,String>();
Example
package Mypkg; import java.util.Hashtable; import java.util.Iterator; import java.util.Map; import java.util.Set; public class MAPex { /** * @param args */ public static void main(String[] args) { // TODO Auto-generated method stub System.out.println("***********************************"); Hashtable<Integer,String> hm=new Hashtable<Integer,String>(); hm.put(100,"Besant"); hm.put(600,"Chennai"); hm.put(200,"Technology"); hm.put(103,"Bangalore"); for(Map.Entry m:hm.entrySet()){ System.out.println(m.getKey()+"-> "+m.getValue()); } System.out.println("***********************************"); } }
Output
*********************************
600-> Chennai
103-> Bangalore
200-> Technology
100-> Besant
***********************************
Click Here-> Get Prepared for Java Interviews
Methods of Hashtable
Method name | Description |
void clear() | Removes all the key-value pairs. |
boolean containsKey(Object key) | Returns true, if the Hashtable contains a value for the specified key. |
boolean contains value(Object value) | Returns true, if the Hashtable maps a value for the specified key. |
Enumeration<V> elements() | Iterates the values in the Hashtable. |
Object get(Object key) | Returns the value to which the specified key is mapped, or null if the map contains no mapping for the key. |
Set entrySet() | Returns a Sets which contains the key-value pairs of the map. |
int hashCode() | Returns the hash code value for the Hashtable |
boolean isEmpty() | Tests if this Hashtable maps no key value pairs. |
Enumeration<K> keys() | Returns the keys mapped in the Hashtable with the type Enumeration. |
Set<K> keySet() | Returns the keys mapped in the Hashtable as the type Set. |
Value put(K key, V value) | Maps the specified key with the specified value in the Hashtable. |
void rehash() | Increases the size of the Hashtable and re-maps the key values according to the new size. |
V replace(K key, V value) | Replaces the value of the specified key with the value specified. |
int size() | Returns the number of key-value pairs in the map. |
Value remove(Object key) | Removes the key value pair for the specified key from the map if it is present. |
Click Here-> Get Java Training with Real-time Projects