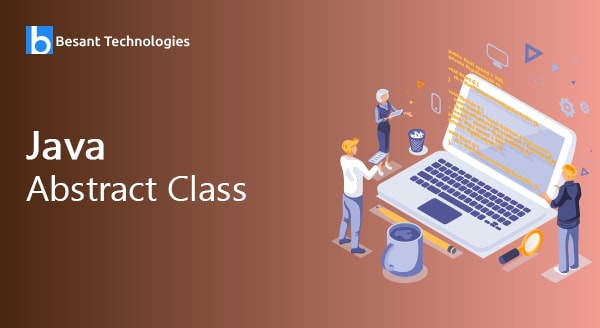
Abstract class in Java
Abstract class in Java
- Abstraction is a process of hiding the implementation details and showing only functionality to the user.
- Abstraction lets you focus on what the object does instead of how it does it
- An abstract class may or may not have an abstract method.
- There is no object creation for Abstract class. It should only be implemented and used by the subclass.
- A subclass which inherits the abstract class either has to implement all the abstract methods present in the abstract class or declare itself as abstract.
- It is like interface but it can have other method definitions apart from abstract method.
- Abstract method is a method which has no implementation only declaration.
abstract void method();
Example:
package Mypkg; public class myabs { /** * @param args */ public static void main(String[] args) { // TODO Auto-generated method stub System.out.println("Entered myabs-Main() "); System.out.println("***************************************"); useabs ob=new useabs(); ob.getDisplay(); ob.getD(); System.out.println("***************************************"); System.out.println("Exited myabs-Main() "); } } abstract class myabsEx { abstract void getDisplay(); // Abstract method void getD() // other method { System.out.println("This is normal method inside Abstract class"); } } class useabs extends myabsEx { void getDisplay() { System.out.println("This is re Definition given of Abstarct method class"); } }
Output :
Entered myabs-Main()
***************************************
This is re Definition given of Abstarct method class
This is normal method inside Abstract class
***************************************
Exited myabs-Main()
Click Here-> Get 100% Practical Java Training